Improving the efficiency of a Python web scraper involves optimizing various aspects of the scraping process, from making HTTP requests to parsing the retrieved data. Here are several strategies:
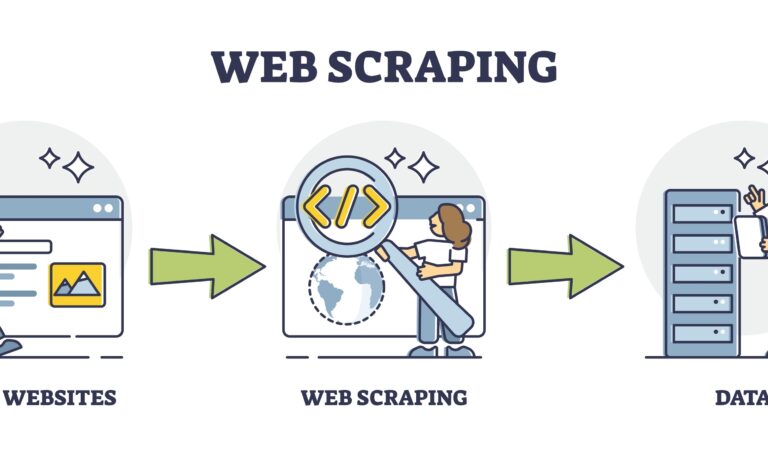
1. **Minimize HTTP Requests**
– **Avoid Unnecessary Requests:** Only request the pages you need. Use URL patterns and parameters to limit the scope of your scraping.
– **Use HTTP Caching:** Implement caching to avoid fetching the same page multiple times.
– **Leverage API Endpoints:** If the website offers an API, use it instead of scraping HTML, as APIs are typically more efficient and less likely to change.
2. **Optimize Request Handling**
– **Asynchronous Requests:** Use libraries like `aiohttp` or `httpx` to make asynchronous requests, allowing your scraper to handle multiple requests concurrently.
– **Batch Requests:** When appropriate, batch multiple requests together to reduce the number of individual HTTP transactions.
3. **Efficient Data Parsing**
– **Selective Parsing:** Only parse the parts of the HTML you need. Use libraries like `BeautifulSoup`, `lxml`, or `selectolax` to efficiently navigate and extract data.
– **XPath/CSS Selectors:** Use precise XPath or CSS selectors to quickly locate and extract data.
4. **Concurrency and Parallelism**
– **Threading:** Use the `concurrent.futures.ThreadPoolExecutor` to manage multiple threads for I/O-bound tasks.
– **Multiprocessing:** Use the `concurrent.futures.ProcessPoolExecutor` for CPU-bound tasks that can benefit from parallel processing.
– **Asyncio:** Combine `asyncio` with `aiohttp` for non-blocking, asynchronous network I/O.
5. **Rate Limiting and Throttling**
– **Respect Robots.txt:** Always check and respect the `robots.txt` file of the website to understand the allowed scraping limits.
– **Rate Limiting:** Implement rate limiting to avoid overwhelming the server and reduce the chance of being blocked.
– **Backoff Strategies:** Use exponential backoff strategies when encountering rate limits or errors.
6. **Data Storage Optimization**
– **Efficient Data Structures:** Use efficient data structures to store and process the scraped data.
– **Incremental Storage:** Save data incrementally to avoid losing progress and reduce memory usage.
7. **Error Handling and Robustness**
– **Retry Logic:** Implement retry logic for transient errors (e.g., network timeouts).
– **Exception Handling:** Use comprehensive exception handling to manage different types of errors gracefully.
8. **Network Optimizations**
– **Compression:** Enable HTTP compression (e.g., gzip) to reduce the amount of data transferred.
– **Session Reuse:** Use persistent sessions (e.g., with `requests.Session`) to reuse TCP connections and reduce latency.
By incorporating these strategies, you can significantly enhance the performance, reliability, and scalability of your Python web scraper.
Contact us if you need any web scrapping requirement
Visit : https://aashyatech.com/contact/
hashtag aashyatech hashtag aashyatechsolutions hashtag webscrapping hashtag php hashtag python hashtag mysql